本文共 976 字,大约阅读时间需要 3 分钟。
本节我们介绍一下在小程序开发中特别常用的功能,布局。介绍了布局的概念和使用方法。
什么是布局
布局是在网页或者移动端开发的一个常见的技术概念,它指的是页面的结构,我们可以把布局理解为在word中的表格,表格分为行和列,在行和列中又可以放置不同的元素,其实日常中有很多场景,我们只要找一些场景的场景分析一下就可以,比如我经常喜欢看腾讯网,那么我们就分析一下腾讯网首页的布局。

如果我们用布局的思维就可以用网格线标一下

标好之后我们可以分析一下,他其实是四行布局,前三行都是一行一列布局,到了第四行的时候是一行三列布局
低代码中的布局组件
低代码码中也有行列布局,行代表Row,列代表Col。但是手机屏幕比较小,每一列占多少像素呢?为了简便,低代码中把页面的宽度定义为12,可以具体设置每一列的宽度,而且可以设置列的宽度
实战
我们今天使用布局组件实现一个教师列表的展示信息,内容包含教师的图片,教师的名称,教师的编号,教龄,所教科目,所属地区等信息。
我们先放置一行,点击row组件
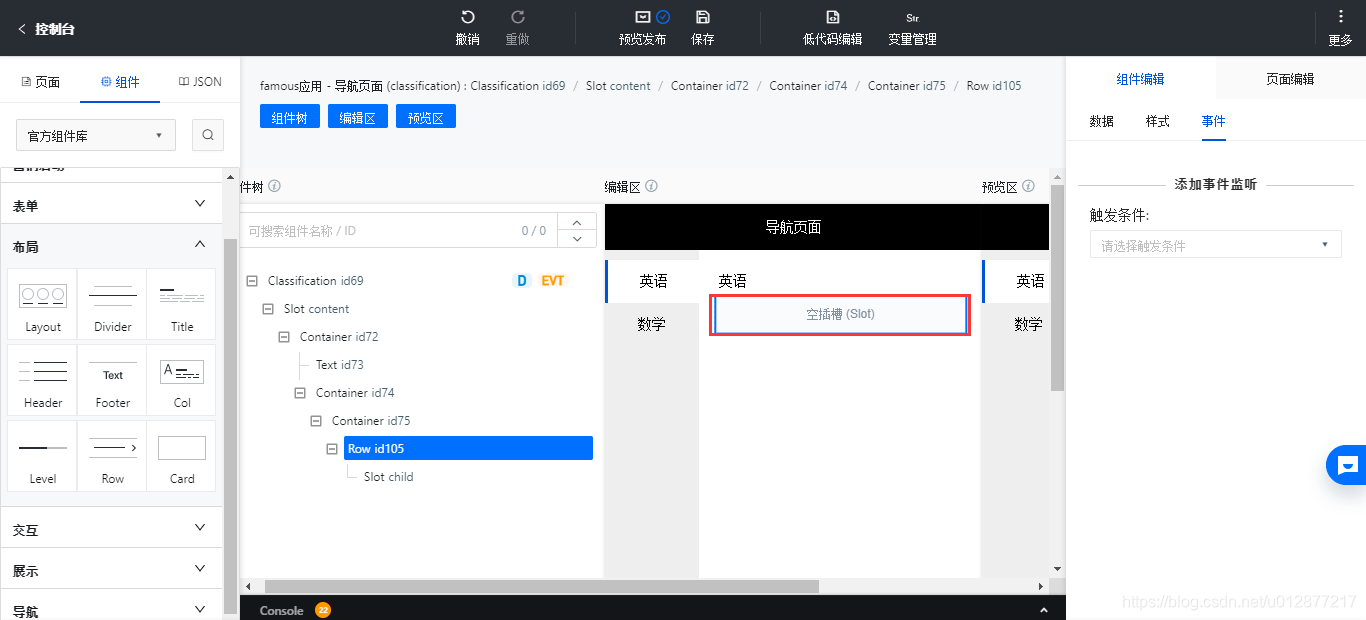
刚了解的网友可能要问,这个空插槽是什么意思,其实打个形象的比方它就像我们电脑的主板一样,上面有很多插槽,可以插内存条。插槽就代表这个组件是容器,可以放置其他的组件。
我们在组件树选中Row的Slot插槽,然后增加两个col组件
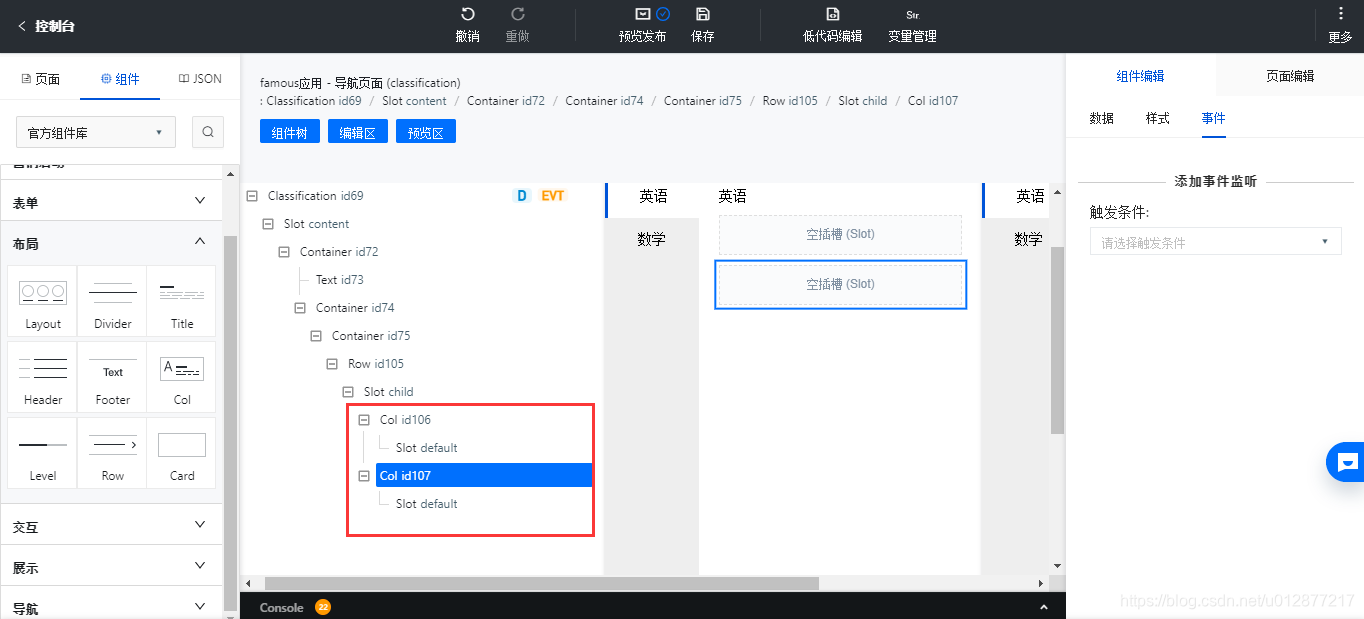
第一个col我们在插槽增加image组件,设置方法同上。
默认图片的大小是占满屏幕,我们可以设置一下图片的大小,在组件树上选中image组件,然后切换到样式标签,设置一下宽和高
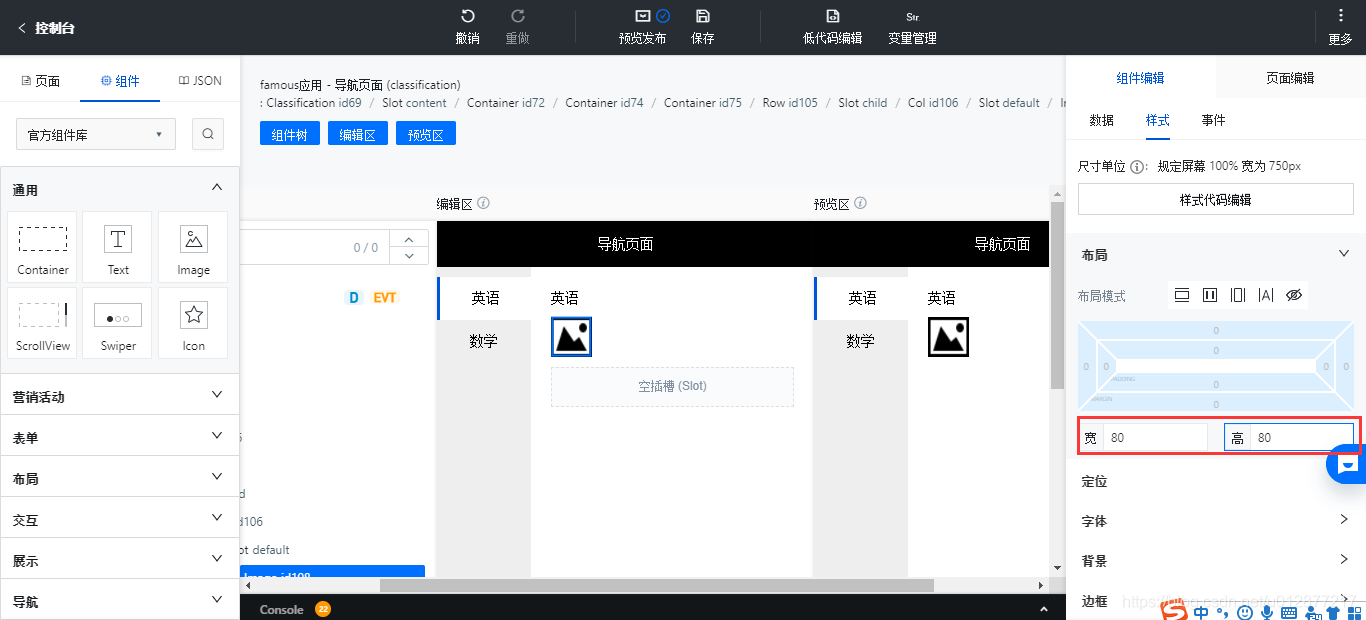
选中第二行的slot插槽,增加三个row组件
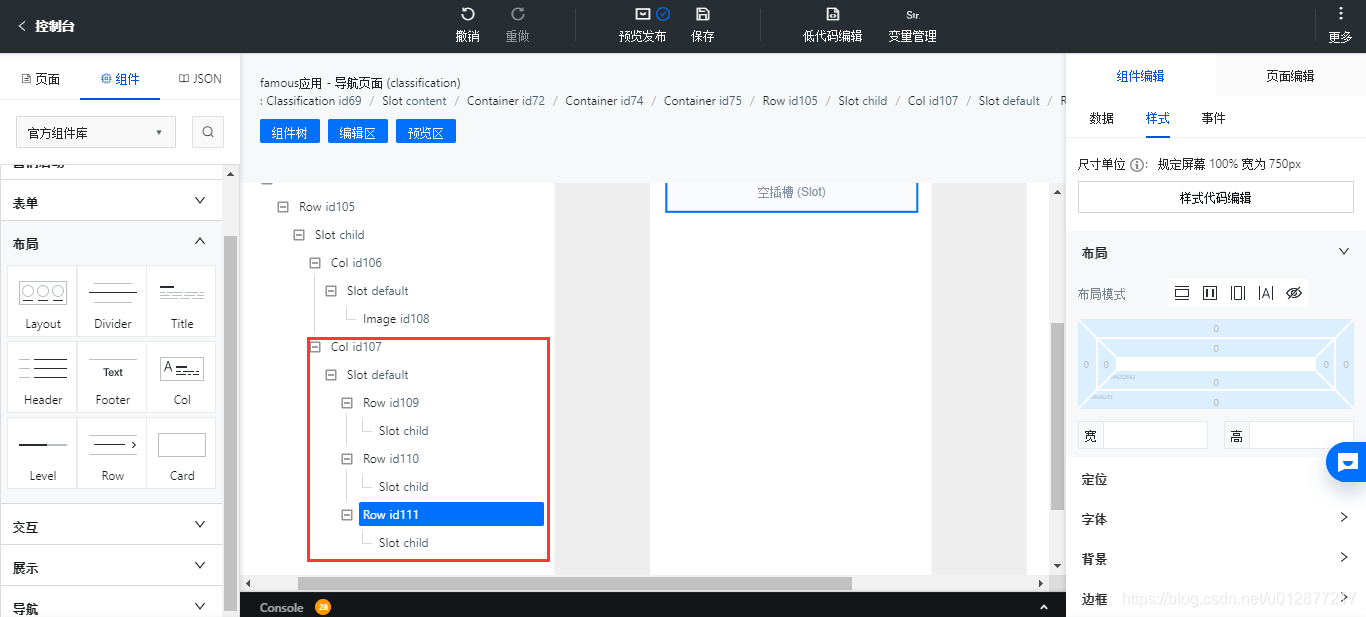
然后每个row增加一个col组件
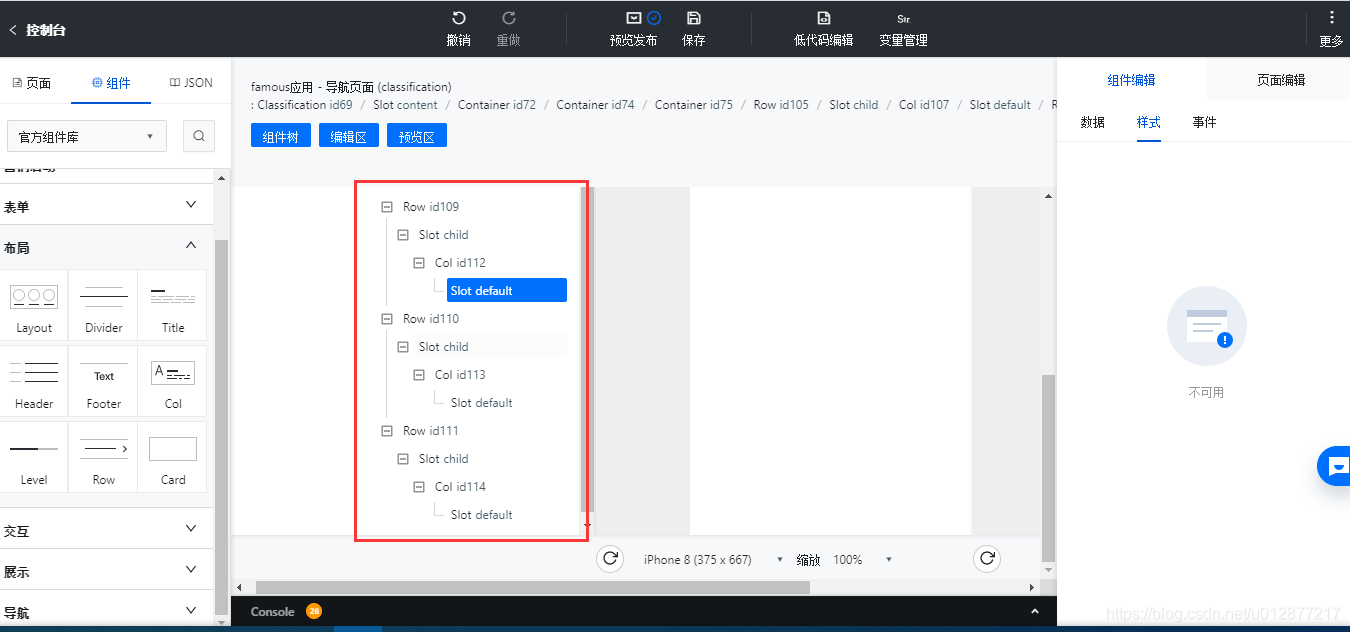
最后在每一列中放置text组件来表示具体的信息
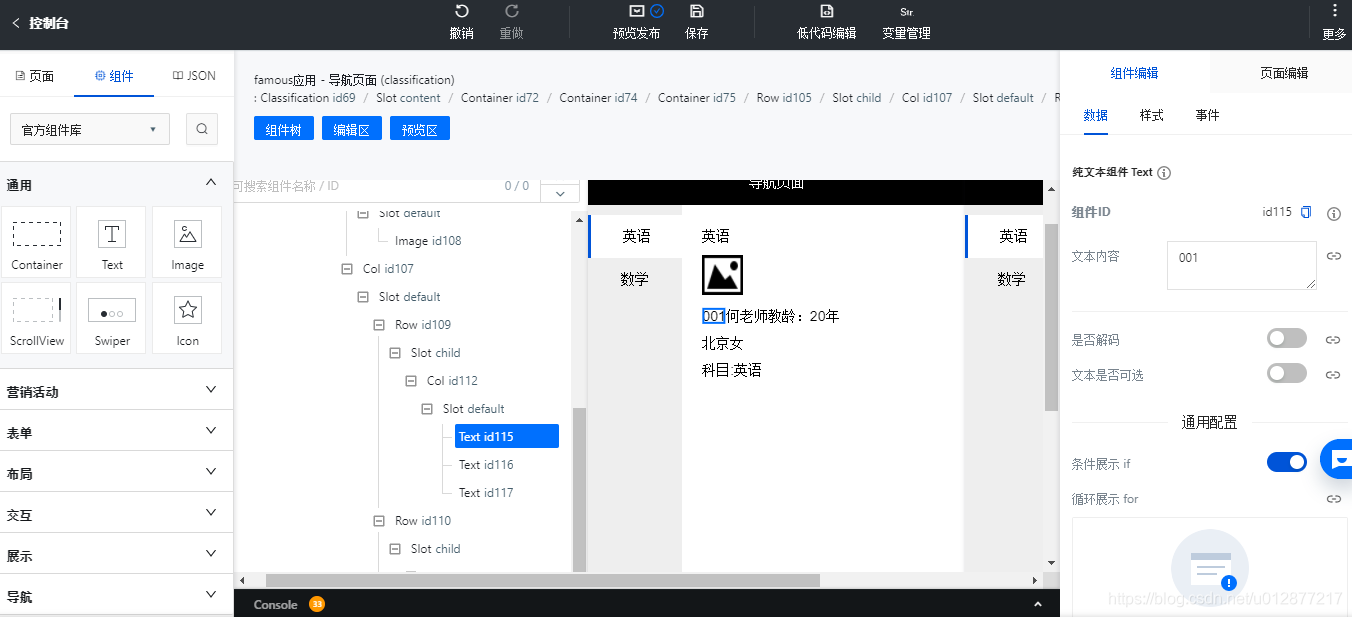
组件添加完成之后就需要设置col的布局,我们先设置最外边的两列,第一列的class属性选择col-3,第二列的class属性选择col-9
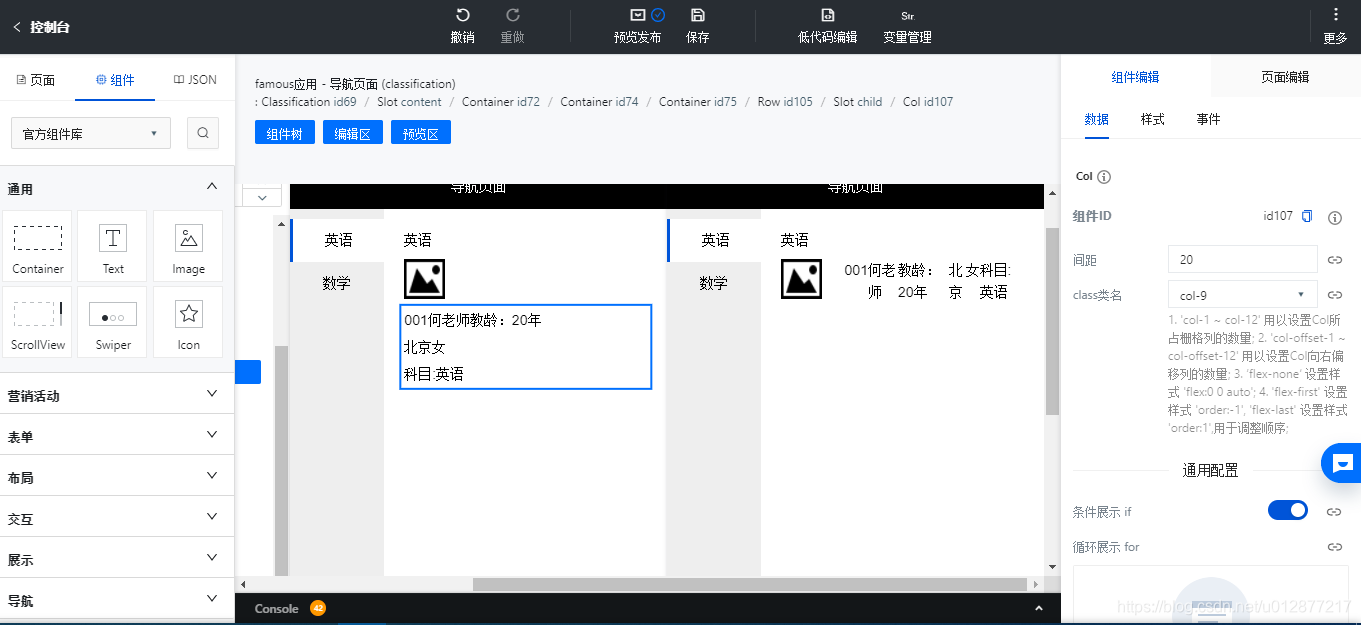
设置好后可能不是你需要的效果,调样式的技巧就是打开元素的边框,然后看为啥没占满,比如打开col组件的边框,设置方法是在样式中打开边框的配置,选择实线,然后选择颜色为黑色,设置边框的宽度为1
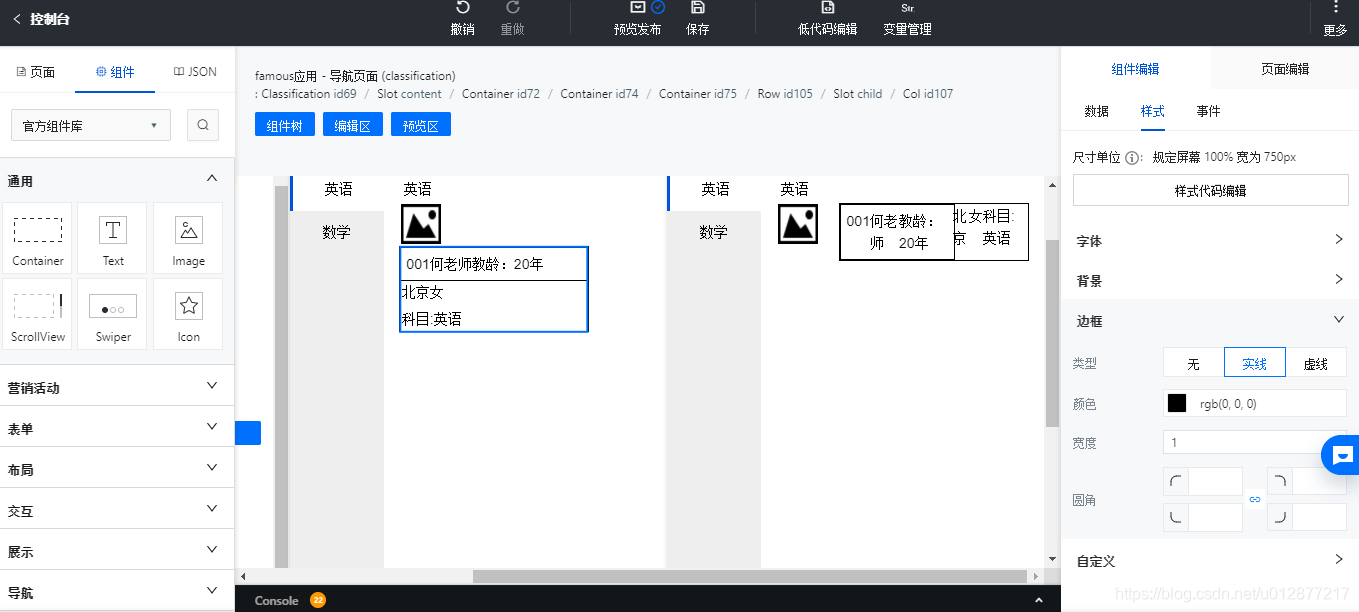
边框一打开你就明白了,剩下的细节就需要仔细调整了,我们现在的问题是列里增加了三行,期望的效果是从上到下排列,但是却挤到了一起,所以需要设置一下列的布局模式,修改为flex布局,然后主轴设置为垂直就好了
转载地址:http://zibzz.baihongyu.com/